Symfony can generate modules, based on model class definitions from the schema.yml
file, for the back-end of your applications. You can create an entire site administration with only generated administration modules. The examples of this section will describe administration modules added to a backend
application. If your project doesn't have a backend
application, create its skeleton now by calling the generate:app
task:
> php symfony generate:app backend
Administration modules interpret the model by way of a special configuration file called generator.yml
, which can be altered to extend all the generated components and the module look and feel. Such modules benefit from the usual module mechanisms described in previous chapters (layout, routing, custom configuration, autoloading, and so on). You can also override the generated action or templates, in order to integrate your own features into the generated administration, but generator.yml
should take care of the most common requirements and restrict the use of PHP code only to the very specific.
Note Even if most common requirements are covered by the generator.yml
configuration file, you can also configure an administration module via a configuration class as we will see later in this chapter.
14.2.1. Initiating an Administration Module
With symfony, you build an administration on a per-model basis. A module is generated based on a Propel object using the propel:generate-admin
task:
> php symfony propel:generate-admin backend Article
This call is enough to create an article
module in the backend
application based on the Article
class definition, and is accessible by the following:
http://localhost/backend.php/article
The look and feel of a generated module, illustrated in Figures 14-5 and 14-6, is sophisticated enough to make it usable out of the box for a commercial application.
Note The administration modules are based on a REST architecture. The propel:generate-admin
task automatically adds such a route to the routing.yml
configuration file:
# apps/backend/config/routing.yml
article:
class: sfPropelRouteCollection
options:
model: Article
module: article
with_wildcard_routes: true
You can also create your own route and pass the name as an argument to the task instead of the model class name:
> php symfony propel:generate-admin backend article
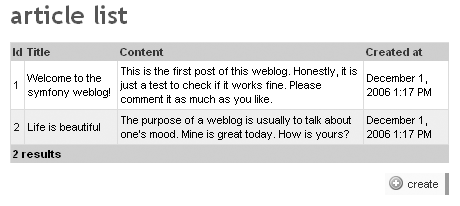
Figure 14.2 list view of the article module in the backend application
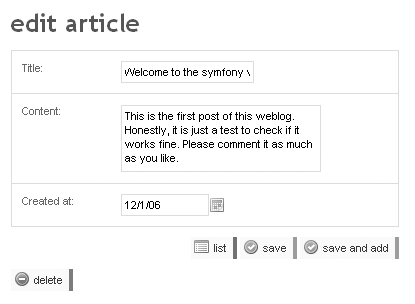
Figure 14.3 edit view of the article module in the backend application
Tip If you don't see the expected look and feel (no stylesheet and no image), this is because you need to install the assets in your project by running the plugin:publish-assets
task:
$ php symfony plugin:publish-assets
14.2.2. A Look at the Generated Code
The code of the Article administration module, in the apps/backend/modules/article/
directory, looks empty because it is only initiated. The best way to review the generated code of this module is to interact with it using the browser, and then check the contents of the cache/
folder. Listing 14-4 lists the generated actions and the templates found in the cache.
Listing 14-4 - Generated Administration Elements, in cache/backend/ENV/modules/article/
// Actions in actions/actions.class.php index // Displays the list of the records of the table filter // Updates the filters used by the list new // Displays the form to create a new record create // Creates a new record edit // Displays a form to modify the fields of a record update // Updates an existing record delete // Deletes a record batch // Executes an action on a list of selected records // In templates/ _assets.php _filters.php _flashes.php _form.php _form_actions.php _form_field.php _form_fieldset.php _form_footer.php _form_header.php _list.php _list_actions.php _list_batch_actions.php _list_field_boolean.php _list_footer.php _list_header.php _list_td_actions.php _list_td_batch_actions.php _list_td_stacked.php _list_td_tabular.php _list_th_stacked.php _list_th_tabular.php _pagination.php editSuccess.php indexSuccess.php newSuccess.php
This shows that a generated administration module is composed mainly of three views, list
, new
, and edit
. If you have a look at the code, you will find it to be very modular, readable, and extensible.
14.2.3. Introducing the generator.yml Configuration File
The generated administration modules rely on parameters found in the generator.yml
YAML configuration file. To see the default configuration of a newly created administration module, open the generator.yml
file, located in the backend/modules/article/config/generator.yml
directory and reproduced in Listing 14-5.
Listing 14-5 - Default Generator Configuration, in backend/modules/article/config/generator.yml
generator:
class: sfPropelGenerator
param:
model_class: BlogArticle
theme: admin
non_verbose_templates: true
with_show: false
singular: ~
plural: ~
route_prefix: article
with_propel_route: 1
config:
actions: ~
list: ~
filter: ~
form: ~
edit: ~
new: ~
This configuration is enough to generate the basic administration. Any customization is added under the config
key. Listing 14-6 shows a typical customized generator.yml
.
Listing 14-6 - Typical Complete Generator Configuration
generator:
class: sfPropelGenerator
param:
model_class: BlogArticle
theme: admin
non_verbose_templates: true
with_show: false
singular: ~
plural: ~
route_prefix: article
with_propel_route: 1
config:
actions:
_new: { label: "Create a new Article", credentials: editor }
fields:
author_id: { label: Article author }
published_on: { credentials: editor }
list:
title: Articles
display: [title, author_id, category_id]
fields:
published_on: { date_format: dd/MM/yy }
layout: stacked
params: |
%%is_published%%<strong>%%=title%%</strong><br /><em>by %%author%%
in %%category%% (%%published_on%%)</em><p>%%content_summary%%</p>
max_per_page: 2
sort: [title, asc]
filter:
display: [title, category_id, author_id, is_published]
form:
display:
"Post": [title, category_id, content]
"Workflow": [author_id, is_published, created_on]
fields:
published_at: { help: "Date of publication" }
title: { attributes: { style: "width: 350px" } }
new:
title: New article
edit:
title: Editing article "%%title%%"
In this configuration, there are six sections. Four of them represent views (list
, filter
, new
, and edit
) and two of them are "virtuals" (fields
and form
) and only exists for configuration purpose.
The following sections explain in detail all the parameters that can be used in this configuration file.